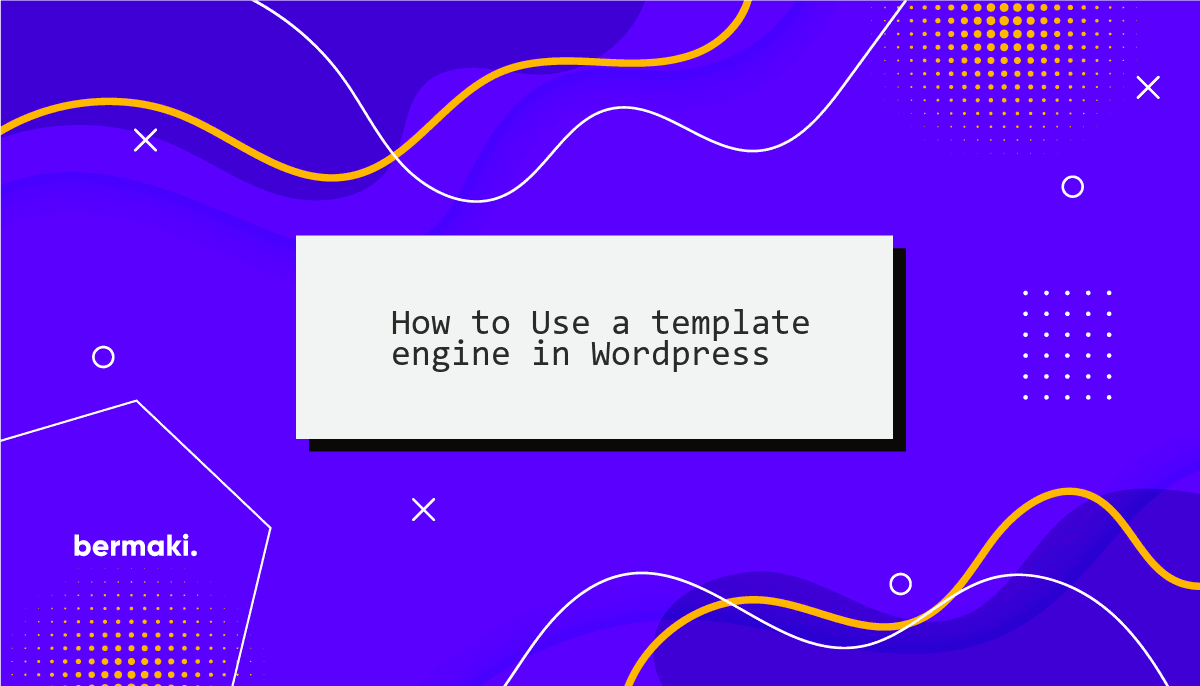
While i'm mostly working with Drupal, i do get some WordPress tasks from time to time. I'm not a big fan of Wordpress, but sometimes you gotta do what you gotta do.
In this post, i want to show you how to use Twig as a template engine in Wordpress. I'm not going to explain how to install Wordpress, but i will show you how to install Twig and use it in your theme.
We will be creating a custom plugin that creates Custom templates that use Twig as a template engine.
What's a template engine?
A template engine is a tool that allows you to separate your logic from your presentation. It's a way to keep your code clean and maintainable. It's also a way to make your code more readable and easier to understand.
Why use Twig?
Twig is a template engine that is used by many popular CMSs, such as Drupal it's also used by many popular frameworks, such as Symfony. It's a very powerful and flexible template engine that allows you to write clean and maintainable code.
How to use Twig in Wordpress
We will be using Timber to integrate Twig into Wordpress. Timber is a Wordpress plugin that allows you to use Twig as a template engine in Wordpress.
Step1: Create a plugin
Head to your plugins directory and create a new directory called twig-plugin
. Inside that directory, create a new file called twig-plugin.php
. This is the main file of our plugin.
Step2: Create a composer.json file
Inside the twig-plugin
directory, create a new file called composer.json
. This file will contain all the dependencies that our plugin needs. We will be using composer to install these dependencies.
{
"require": {
"timber/timber": "^1.23",
},
"config": {
"allow-plugins": {
"composer/installers": true
}
}
}
Step 3: Install the dependencies
Now that we have our composer.json
file, we can install the dependencies using composer. If you don't have composer installed, you can download it from here.
composer install
Step 4: Create the plugin code
Now that we have our plugin installed, we can activate it and create a template. To activate the plugin, go to the plugins page and click on the activate link next to the plugin name.
Once the plugin is activated, we need to create the code that will allow us to register custom templates and use them in our theme.
<?php
/*
Plugin Name: Plugin Twig
Description: Creates Template pages using Twig
Version: 1.0.0
Author: Babel Group
Author URI: https://www.bermaki.com/
License: GPLv2 or later
License URI: https://www.gnu.org/licenses/gpl-2.0.html
Text Domain: plugin-twig
*/
require_once ABSPATH . '/vendor/autoload.php';
function my_template_array() {
$temps = [];
$temps['my-template.php'] = __( 'My Template Name', 'plugin-twig' );
return $temps;
}
function my_template_register( $page_templates, $theme, $post ) {
$templates = my_template_array();
foreach ( $templates as $tk => $tv ) {
$page_templates[ $tk ] = $tv;
}
return $page_templates;
}
add_filter( 'theme_page_templates', 'my_template_register', 10, 3 );
function my_template_select( $template ) {
global $post, $wp_query, $wpdb;
$page_temp_slug = get_page_template_slug( $post->ID );
$custom_template = plugin_dir_path( __FILE__ ) . 'templates/' . $page_temp_slug;
// Check if the custom template exists in the theme directory
if ( $page_temp_slug && file_exists( $custom_template ) ) {
return $custom_template;
}
return $template;
}
add_filter( 'template_include', 'my_template_select', 99 );
Let me break down what each part of the code does:
my_template_array()
This function creates an associative array called $temps containing template file names as keys and corresponding translated names as values. These templates can then be used in the WordPress admin to assign a custom template to a page.
my_template_register()
This function takes an array of existing page templates ($page_templates), the current theme ($theme), and a post object ($post). It uses the my_template_array()
function to get a list of custom template file names and their translated names. It then adds these custom templates to the $page_templates array and returns the modified array.
add_filter( 'theme_page_templates', 'my_template_register', 10, 3 )
This line hooks the my_template_register
function into the theme_page_templates
filter. When WordPress processes page templates, this filter allows custom templates to be added to the list of available templates.
my_template_select()
This function checks if a custom template file exists in the theme directory. It first gets the slug of the current page template being used. Then, it constructs the path to the custom template file inside a templates
directory within the plugin. If the custom template file exists, it returns the path to that file. If not, it falls back to the original template.
add_filter( 'template_include', 'my_template_select', 99 );
This line hooks the my_template_select
function into the template_include filter. This filter allows the plugin to override the template used to display a WordPress page. In this case, the function checks for the existence of a custom template and uses it if available, or falls back to the default template.
Step5: Create a template file
- My templates files are located in
twig-plugin/templates/
directory. - The template files are named after the template name that we registered in the
my_template_array()
function.
Here's an example of a template file:
<?php
/**
* Template Name: My Template Name
*/
get_header();
use Timber\Timber;
$context = Timber::get_context();
$context['myvar'] = 'Hello World';
Timber::render( 'my-template.twig', $context );
get_footer();
We Use Timber to render the template. Timber::render expects two parameters, the first one is the template file name and the second one is the context array. The context array contains all the variables that we want to pass to the template, in our case we are passing a variable called myvar
that contains the string Hello World
.
Step 6: Create a Twig file
My twig file will be located in twig-plugin/templates/views
directory. The twig file name is the same as the template file name. This way we can keep everything organized.
<h2>{{ myvar }}</h2>
As simple as that, we can now use Twig in our Wordpress theme.
A more MVC approach
Think of the PHP file as a controller, and the Twig file as a view. The PHP file is responsible for getting the data and passing it to the view. The view is responsible for displaying the data.
As an example, i'd be using php template file like this:
get_header();
use Timber\Timber;
$myHelper = new HelperClass();
$context = Timber::context();
if ( ! empty( $_POST ) ) {
$new_date = "";
if ( ! empty( $_POST['date'] ) ) {
$new_date = date( 'd/m/Y', strtotime( $_POST['date'] ) );
}
// EDITAR
if ( isset( $_GET['id'] ) ) {
$myHelper->changeDate( $_POST, $new_date );
} else {
$myHelper->createDate( $_POST, $new_date );
}
}
$context['btnText'] = 'Save';
Timber::render( 'my-tempalte.twig', $context );
get_footer();
And That's it for today! I hope you found this helpful post. If you have any questions, please contact me on LinkedIn.
Happy coding!